The Rolling Stones - Respectable 11. The Rolling Stones - Far Away Eyes 12. Rolling stones love you live remastered rar. The Rolling Stones - Love In Vain 13. The Rolling Stones - Imagination 09. The Rolling Stones - Shattered 10.
Stack is a subclass of Vector that implements a standard last-in, first-out stack. Stack only defines the default constructor, which creates an empty stack. Stack includes all the methods defined by Vector, and adds several of its own. To put an object on the top of the stack, call push(). To remove and return the top element, call pop(). Java Stack extends Vector class with the following five operations only. Boolean empty(): Tests if this stack is empty. E peek(): Looks at the object at the top of this stack without removing it from the stack. E pop(): Removes the object at the top of this stack and returns that object as the value of this function. Stack is a data structure used in programming. It consists of adding entries from top and removing elements from the top. It is based on concept of LIFO(Last In.
As usual, revewing a design rather than code. As coded, pop takes time proportional to the current stack size. It could and should take constant time. Hint: reverse the semantics of node.next (BTW, first becomes redundant). I don't think that pop/peek returning -1 on empty stack is a good idea. A value of -1 is a valid result of popping from a stack of integers.
Why not return null? Throwing an exception is also a viable option. A client of this implementation cannot use a 2-argument constructor ( class Node is private). Therefore it doesn't have a right to exist. Any value that could be misinterpreted as a valid entry in the stack (like -1) is not a useful error code. If i can't easily tell the difference between legitimately popping a value and underflowing the stack, that's a design error.
Null isn't currently an option either, since the stack allows it to be pushed. (It could be, though, if push(null) threw an IllegalArgumentException or something. But then you're already throwing exceptions; might as well throw a 'stack underflow' exception as well.) – Jun 6 '14 at 7:05. I would look at this code and think you hadn't messed with Java since before 1.5.

You should really be using generics, particularly for a collection-type class like a stack. Any value you allow to be pushed onto the stack, is useless as an error code. Consider the following code: Stack s = new Stack; s.push(-1). Do lots of pushes and pops.
Object value = s.pop; Did i just get an error, or legitimately pop a -1? Either prevent the pushing of null and use that as an error code, or throw an exception if there's nothing to pop. The latter is usually preferable. In addition to the design problems mentioned by @vnp: Any algorithm using a stack contains a while the stack is not empty somewhere, so you have to have a public boolean isEmpty method.
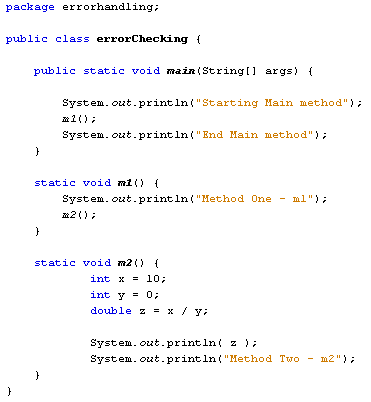
Unless you disallow null values, returning null as a result code is not acceptable, even then inadvisable. You should throw some relevant RuntimeException (as suggested by @Aaronaught), preferebly from the standard libraries: e.g. NoSuchElementException or EmptyStackException etc.
Implement Stack In Java
If you don't have Java reference documentation available, don't know the modus operandi, if it is a whiteboard interview for example, you can throw IllegalStateException. (Every Java programmer should know basic OOP exceptions: IllegalArgumentException, IllegalStateException. Most important, I won't hire someone who claims to be a Java programmer unless he knows generics and uses them properly. This is 10-year-old technology, people. Get with the program.
Second, using '-1' as an error return is just dumb. What if someone pushes -1? Use null (and forbid pushing it). Third, why are you keeping track of the bottom of the stack? Seems like a waste of effort, unless I'm missing something.
Finally, the way you are implementing pop is just bananas. The second most common call, and it is O(n)? A stack is a single-linked list, with only a few methods implemented.
Think about that.
Output of Java Stack Program System.out.println(“Calling empty before elements are added: ” + st.empty); The empty method returns true if no elements exist in the stack. As no elements are added still, the above statement returns true. We know Stack is a legacy class and originally not part of collections framework. As Stack extends Vector and indirectly List also, Stack can make use of all the methods of Collection interface. Add is a method of Collection interface inherited by Stack. The original method of adding, defined in Stack class, is push. Both methods are used in the program.
This is how, the designers, gave compatibility to legacy classes with collection classes. Following is the hierarchy of Stack. Collection – List – Vector – Stack System.out.println(“Index number of A counted from top: ” + st.search(50)); System.out.println(“Index number of SNRao from top: ” + st.search(“SNRao”)); Using search method, the programmer can know at what position or index number, a particular element exist in the stack. The counting starts from the top element (added last). As 50 is added last, serach(50) returns 1. Search('SNRao') returns 6.
Observe the screenshot. System.out.println(“Element at the top: ” + st.peek); The peek method returns the element at the top; that is 50. But the element is not removed from the stack. Observe the code, 50 is again printed with pop method. Of elements before pop called: ” + st.size); The size method returns the number of elements present in the stack.
The same size method when called after pop, prints one less, 8 (original number is 9). System.out.println(“Popped element: ” + st.pop); The pop method returns the top element. The element is permanently comes out and cannot be obtained again. Observe when size is called after while loop, it prints 0 as all the elements are removed from stack with iteration using pop method; now stack is empty. Rewrite this Java stack program with different iterations to get command over the subject. Real-time Examples of Stack Implementations Following are a few examples of Stack in realtime.
Cement bags in a godown. Clothes in an almyrah.
Bullets loaded in a gun. With this knowledge of general Java stack program, now you can understand the second Java Stack program,. Pass your comments to improve the quality of this tutorial' Java Stack Program'.